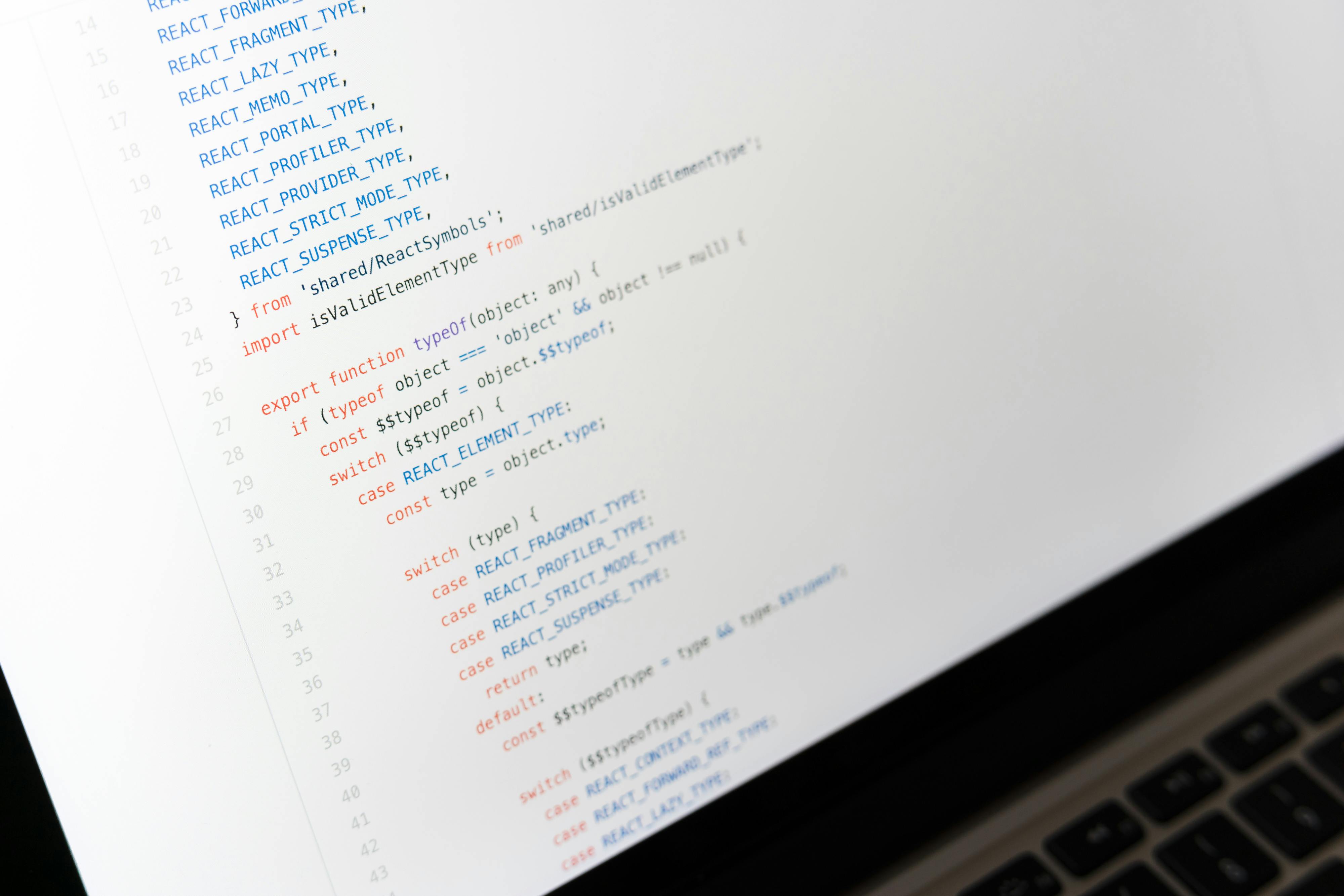
Why TypeScript is Worth Learning
TypeScript adds static typing to JavaScript, providing better tooling, more robust code, and improved developer experience.
Gerrad Zhang
Wuhan, China
1 min read
Introduction to TypeScript
TypeScript has become an essential tool in modern web development. As a superset of JavaScript, it adds optional static typing and other features that make code more maintainable and easier to understand.
Key Benefits
1. Type Safety
TypeScript’s main feature is its type system. This helps catch errors early in development:
function greet(name: string) {
return `Hello, ${name}!`;
}
// This will error during compilation
greet(123); // Error: Argument of type 'number' is not assignable to parameter of type 'string'
2. Better IDE Support
With TypeScript, you get:
- Improved autocomplete
- Better refactoring tools
- Inline documentation
- Real-time error detection
3. Enhanced Code Quality
TypeScript helps maintain code quality through:
- Interface definitions
- Type checking
- Code organization
- Clear dependencies
Getting Started
Install TypeScript globally:
npm install -g typescript
Create your first TypeScript file:
interface User {
name: string;
age: number;
}
function createUser(user: User): User {
return user;
}
const newUser = createUser({ name: "John", age: 30 });